by Jesmin Akther | Oct 19, 2021 | C Programming
Conversion from uppercase to lower case using c program
#include<stdio.h>
#include<string.h>
int main(){
char str[20];
int i;
printf("Enter any string->");
scanf("%s",str);
printf("The string is->%s",str);
for(i=0;i<=strlen(str);i++){
if(str[i]>=65&&str[i]<=90)
str[i]=str[i]+32;
}
printf("\nThe string in lower case is->%s",str);
return 0;
}
Algorithm:
ASCII value of ‘A’ is 65 while ‘a’ is 97. Difference between them is 97 – 65 = 32
So if we will add 32 in the ASCII value of ‘A’ then it will be ‘a’ and if will we subtract 32 in ASCII value of ‘a’ it will be ‘A’. It is true for all alphabets.
In general rule:
Upper case character = Lower case character – 32
Lower case character = Upper case character + 32
Write a c program to convert the string from lower case to upper case
#include<stdio.h>
int main(){
char str[20];
int i;
printf("Enter any string->");
scanf("%s",str);
printf("The string is->%s",str);
for(i=0;i<=strlen(str);i++){
if(str[i]>=97&&str[i]<=122)
str[i]=str[i]-32;
}
printf("\nThe string in lowercase is->%s",str);
return 0;
}
Algorithm:
ASCII value of ‘A’ is 65 while ‘a’ is 97. Difference between them is 97 – 65 = 32
So if we will add 32 in the ASCII value of ‘A’ then it will be ‘a’ and if will we subtract 32 in ASCII value of ‘a’ it will be ‘A’. It is true for all alphabets.
In general rule:
Upper case character = Lower case character – 32
Lower case character = Upper case character + 32
COUNTING DIFFERENT CHARACTERS IN A STRING USING C PROGRAM
#include <stdio.h>
int isvowel(char chk);
int main(){
char text[1000], chk;
int count;
count = 0;
while((text[count] = getchar()) != '\n')
count++;
text[count] = '\0';
count = 0;
while ((chk = text[count]) != '\0'){
if (isvowel(chk)){
if((chk = text[++count]) && isvowel(chk)){
putchar(text[count -1]);
putchar(text[count]);
putchar('\n');
}
}
else
++count;
}
return 0;
}
int isvowel(char chk){
if(chk == 'a' || chk == 'e' || chk == 'i' || chk == 'o' || chk == 'u')
return 1;
return 0;
}
Program for sorting of string in c language
#include<stdio.h>
int main(){
int i,j,n;
char str[20][20],temp[20];
puts("Enter the no. of string to be sorted");
scanf("%d",&n);
for(i=0;i<=n;i++)
gets(str[i]);
for(i=0;i<=n;i++)
for(j=i+1;j<=n;j++){
if(strcmp(str[i],str[j])>0){
strcpy(temp,str[i]);
strcpy(str[i],str[j]);
strcpy(str[j],temp);
}
}
printf("The sorted string\n");
for(i=0;i<=n;i++)
puts(str[i]);
return 0;
}
Concatenation of two strings in c programming language
#include<stdio.h>
int main(){
int i=0,j=0;
char str1[20],str2[20];
puts(“Enter first string”);
gets(str1);
puts(“Enter second string”);
gets(str2);
printf(“Before concatenation the strings are\n”);
puts(str1);
puts(str2);
while(str1[i]!=’\0′){
i++;
}
while(str2[j]!=’\0′){
str1[i++]=str2[j++];
}
str1[i]=’\0′;
printf(“After concatenation the strings are\n”);
puts(str1);
return 0;
}
Concatenation of two strings using pointer in c programming language
#include<stdio.h>
int main(){
int i=0,j=0;
char *str1,*str2,*str3;
puts("Enter first string");
gets(str1);
puts("Enter second string");
gets(str2);
printf("Before concatenation the strings are\n");
puts(str1);
puts(str2);
while(*str1){
str3[i++]=*str1++;
}
while(*str2){
str3[i++]=*str2++;
}
str3[i]='\0';
printf("After concatenation the strings are\n");
puts(str3);
return 0;
}
C code which prints initial of any name
#include<stdio.h>
int main(){
char str[20];
int i=0;
printf("Enter a string: ");
gets(str);
printf("%c",*str);
while(str[i]!='\0'){
if(str[i]==' '){
i++;
printf("%c",*(str+i));
}
i++;
}
return 0;
}
Sample output:
Enter a string: Robert De Niro
RDN
Write a c program to print the string from given character
#include<string.h>
#include<stdio.h>
int main(){
char *p;
char s[20],s1[1];
printf("\nEnter a string: ");
scanf("%[^\n]",s);
fflush(stdin);
printf("\nEnter character: ");
gets(s1);
p=strpbrk(s,s1);
printf("\nThe string from the given character is: %s",p);
return 0;
}
Reverse a string in c without using temp
String reverse using strrev in c programming language
#include<stdio.h>
#include<string.h>
int main(){
char str[50];
char *rev;
printf("Enter any string : ");
scanf("%s",str);
rev = strrev(str);
printf("Reverse string is : %s",rev);
return 0;
}
String reverse in c without using strrev
String reverse in c without using string function
How to reverse a string in c without using reverse function
#include<stdio.h>
int main(){
char str[50];
char rev[50];
int i=-1,j=0;
printf("Enter any string : ");
scanf("%s",str);
while(str[++i]!='\0');
while(i>=0)
rev[j++] = str[--i];
rev[j]='\0';
printf("Reverse of string is : %s",rev);
return 0;
}
Sample output:
Enter any string : cquestionbank.blogspot.com
Reverse of string is : moc.topsgolb.knabnoitseuqc
C code to reverse a string by recursion:
#include<stdio.h>
#define MAX 100
char* getReverse(char[]);
int main(){
char str[MAX],*rev;
printf("Enter any string: ");
scanf("%s",str);
rev = getReverse(str);
printf("Reversed string is: %s",rev);
return 0;
}
char* getReverse(char str[]){
static int i=0;
static char rev[MAX];
if(*str){
getReverse(str+1);
rev[i++] = *str;
}
return rev;
}
Sample output:
Enter any string: mona
Reversed string is: anom
String concatenation in c without using string functions
#include<stdio.h>
void stringConcat(char[],char[]);
int main(){
char str1[100],str2[100];
int compare;
printf("Enter first string: ");
scanf("%s",str1);
printf("Enter second string: ");
scanf("%s",str2);
stringConcat(str1,str2);
printf("String after concatenation: %s",str1);
return 0;
}
void stringConcat(char str1[],char str2[]){
int i=0,j=0;
while(str1[i]!='\0'){
i++;
}
while(str2[j]!='\0'){
str1[i] = str2[j];
i++;
j++;
}
str1[i] = '\0';
}
Sample output:
Enter first string: cquestionbank
Enter second string: @blogspot.com
String after concatenation: [email protected]
C program to compare two strings without using string functions
#include<stdio.h>
int stringCompare(char[],char[]);
int main(){
char str1[100],str2[100];
int compare;
printf("Enter first string: ");
scanf("%s",str1);
printf("Enter second string: ");
scanf("%s",str2);
compare = stringCompare(str1,str2);
if(compare == 1)
printf("Both strings are equal.");
else
printf("Both strings are not equal");
return 0;
}
int stringCompare(char str1[],char str2[]){
int i=0,flag=0;
while(str1[i]!='\0' && str2[i]!='\0'){
if(str1[i]!=str2[i]){
flag=1;
break;
}
i++;
}
if (flag==0 && str1[i]=='\0' && str2[i]=='\0')
return 1;
else
return 0;
}
Sample output:
Enter first string: cquestionbank.blogspot.com
Enter second string: cquestionbank.blogspot.com
Both strings are equal.
String copy without using strcpy in c programming language
#include<stdio.h>
void stringCopy(char[],char[]);
int main(){
char str1[100],str2[100];
printf("Enter any string: ");
scanf("%s",str1);
stringCopy(str1,str2);
printf("After copying: %s",str2);
return 0;
}
void stringCopy(char str1[],char str2[]){
int i=0;
while(str1[i]!='\0'){
str2[i] = str1[i];
i++;
}
str2[i]='\0';
}
Sample output:
Enter any string: cquestionbank.blogspot.com
After copying: cquestionbank.blogspot.com
Program to convert string into ASCII values in c programming language:
#include<stdio.h>
int main(){
char str[100];
int i=0;
printf("Enter any string: ");
scanf("%s",str);
printf("ASCII values of each characters of given string: ");
while(str[i])
printf("%d ",str[i++]);
return 0;
}
Sample Output:
Enter any string: cquestionbank.blogspot.com
ASCII values of each characters of given string: 99 113 117 101 115 116 105 111 110 98 97 110 107 46 98 108 111 103 115 112 111 116 46 99 111 109
by Jesmin Akther | Aug 29, 2021 | Artificial Intelligence
What is Artificial Intelligence?
AI has the potential to help humans live more meaningful lives that are devoid of hard labour. According to the father of Artificial Intelligence, John McCarthy, it is “The science and engineering of making intelligent machines, especially intelligent computer programs”.
Artificial Intelligence is a way of making a computer, a computer-controlled robot, or a software think intelligently, in the similar manner the intelligent humans think. AI is accomplished by studying how human brain thinks, and how humans learn, decide, and work while trying to solve a problem, and then using the outcomes of this study as a basis of developing intelligent software and systems.
Goals of AI
- To Create Expert Systems − The systems which exhibit intelligent behavior, learn, demonstrate, explain, and advice its users.
- To Implement Human Intelligence in Machines − Creating systems that understand, think, learn, and behave like humans.
Programming Without and With AI
The programming without and with AI is different in following ways −
Programming Without AI |
Programming With AI |
A computer program without AI can answer the specific questions it is meant to solve. |
A computer program with AI can answer the generic questions it is meant to solve. |
Modification in the program leads to change in its structure. |
AI programs can absorb new modifications by putting highly independent pieces of information together. Hence you can modify even a minute piece of information of program without affecting its structure. |
Modification is not quick and easy. It may lead to affecting the program adversely. |
Quick and Easy program modification. |
What is AI Technique?
In the real world, the knowledge has some unwelcomed properties:
- Its volume is huge, next to unimaginable.
- It is not well-organized or well-formatted.
- It keeps changing constantly.
AI Technique is a manner to organize and use the knowledge efficiently in such a way that:
- It should be perceivable by the people who provide it.
- It should be easily modifiable to correct errors.
- It should be useful in many situations though it is incomplete or inaccurate.
AI techniques elevate the speed of execution of the complex program it is equipped with.
Applications of AI
AI has been dominant in various fields such as:
-
- Gaming − AI plays crucial role in strategic games such as chess, poker, tic-tac-toe, etc., where machine can think of large number of possible positions based on heuristic knowledge.
- Natural Language Processing − It is possible to interact with the computer that understands natural language spoken by humans.
- Expert Systems − There are some applications which integrate machine, software, and special information to impart reasoning and advising. They provide explanation and advice to the users.
- Vision Systems − These systems understand, interpret, and comprehend visual input on the computer. For example,
- A spying aeroplane takes photographs, which are used to figure out spatial information or map of the areas.
- Doctors use clinical expert system to diagnose the patient.
- Police use computer software that can recognize the face of criminal with the stored portrait made by forensic artist.
- Speech Recognition − Some intelligent systems are capable of hearing and comprehending the language in terms of sentences and their meanings while a human talks to it. It can handle different accents, slang words, noise in the background, change in human’s noise due to cold, etc.
- Handwriting Recognition − The handwriting recognition software reads the text written on paper by a pen or on screen by a stylus. It can recognize the shapes of the letters and convert it into editable text.
- Intelligent Robots − Robots are able to perform the tasks given by a human. They have sensors to detect physical data from the real world such as light, heat, temperature, movement, sound, bump, and pressure. They have efficient processors, multiple sensors and huge memory, to exhibit intelligence. In addition, they are capable of learning from their mistakes and they can adapt to the new environment.
[wpsbx_html_block id=1891]
Advantages of Artificial Intelligence
Following are some main advantages of Artificial Intelligence:
- High Accuracy with less errors: AI machines or systems are prone to less errors and high accuracy as it takes decisions as per pre-experience or information.
- High-Speed: AI systems can be of very high-speed and fast-decision making, because of that AI systems can beat a chess champion in the Chess game.
- High reliability: AI machines are highly reliable and can perform the same action multiple times with high accuracy.
- Useful for risky areas: AI machines can be helpful in situations such as defusing a bomb, exploring the ocean floor, where to employ a human can be risky.
- Digital Assistant: AI can be very useful to provide digital assistant to the users such as AI technology is currently used by various E-commerce websites to show the products as per customer requirement.
- Useful as a public utility: AI can be very useful for public utilities such as a self-driving car which can make our journey safer and hassle-free, facial recognition for security purpose, Natural language processing to communicate with the human in human-language, etc.
Disadvantages of Artificial Intelligence
Every technology has some disadvantages, and thesame goes for Artificial intelligence. Being so advantageous technology still, it has some disadvantages which we need to keep in our mind while creating an AI system. Following are the disadvantages of AI:
-
- High Cost: The hardware and software requirement of AI is very costly as it requires lots of maintenance to meet current world requirements.
- Can’t think out of the box: Even we are making smarter machines with AI, but still they cannot work out of the box, as the robot will only do that work for which they are trained, or programmed.
- No feelings and emotions: AI machines can be an outstanding performer, but still it does not have the feeling so it cannot make any kind of emotional attachment with human, and may sometime be harmful for users if the proper care is not taken.
- Increase dependency on machines: With the increment of technology, people are getting more dependent on devices and hence they are losing their mental capabilities.
- No Original Creativity: As humans are so creative and can imagine some new ideas but still AI machines cannot beat this power of human intelligence and cannot be creative and imaginative.
What is Intelligence?
The ability of a system to calculate, reason, perceive relationships and analogies, learn from experience, store and retrieve information from memory, solve problems, comprehend complex ideas, use natural language fluently, classify, generalize, and adapt new situations.
What is Intelligence Composed of?
The intelligence is intangible. It is composed of −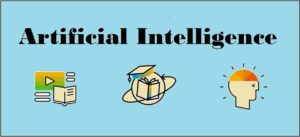
- Reasoning
- Learning
- Problem Solving
- Perception
- Linguistic Intelligence
Let us go through all the components briefly −
- Reasoning − It is the set of processes that enables us to provide basis for judgement, making decisions, and prediction. There are broadly two types −
Inductive Reasoning |
Deductive Reasoning |
It conducts specific observations to makes broad general statements. |
It starts with a general statement and examines the possibilities to reach a specific, logical conclusion. |
Even if all of the premises are true in a statement, inductive reasoning allows for the conclusion to be false. |
If something is true of a class of things in general, it is also true for all members of that class. |
Example − “Nita is a teacher. Nita is studious. Therefore, All teachers are studious.” |
Example − “All women of age above 60 years are grandmothers. Shalini is 65 years. Therefore, Shalini is a grandmother.” |
- Learning − It is the activity of gaining knowledge or skill by studying, practising, being taught, or experiencing something. Learning enhances the awareness of the subjects of the study.The ability of learning is possessed by humans, some animals, and AI-enabled systems. Learning is categorized as −
- Auditory Learning − It is learning by listening and hearing. For example, students listening to recorded audio lectures.
- Episodic Learning − To learn by remembering sequences of events that one has witnessed or experienced. This is linear and orderly.
- Motor Learning − It is learning by precise movement of muscles. For example, picking objects, Writing, etc.
- Observational Learning − To learn by watching and imitating others. For example, child tries to learn by mimicking her parent.
- Perceptual Learning − It is learning to recognize stimuli that one has seen before. For example, identifying and classifying objects and situations.
- Relational Learning − It involves learning to differentiate among various stimuli on the basis of relational properties, rather than absolute properties. For Example, Adding ‘little less’ salt at the time of cooking potatoes that came up salty last time, when cooked with adding say a tablespoon of salt.
- Spatial Learning − It is learning through visual stimuli such as images, colors, maps, etc. For Example, A person can create roadmap in mind before actually following the road.
- Stimulus-Response Learning − It is learning to perform a particular behavior when a certain stimulus is present. For example, a dog raises its ear on hearing doorbell.
- Problem Solving − It is the process in which one perceives and tries to arrive at a desired solution from a present situation by taking some path, which is blocked by known or unknown hurdles.Problem solving also includes decision making, which is the process of selecting the best suitable alternative out of multiple alternatives to reach the desired goal are available.
- Perception − It is the process of acquiring, interpreting, selecting, and organizing sensory information.Perception presumes sensing. In humans, perception is aided by sensory organs. In the domain of AI, perception mechanism puts the data acquired by the sensors together in a meaningful manner.
- Linguistic Intelligence − It is one’s ability to use, comprehend, speak, and write the verbal and written language. It is important in interpersonal communication.
Difference between Human and Machine Intelligence
- Humans perceive by patterns whereas the machines perceive by set of rules and data.
- Humans store and recall information by patterns, machines do it by searching algorithms. For example, the number 40404040 is easy to remember, store, and recall as its pattern is simple.
- Humans can figure out the complete object even if some part of it is missing or distorted; whereas the machines cannot do it correctly.
by Jesmin Akther | Jun 5, 2021 | JAVA
Java Packages & API
In Java, a package is group related classes or a folder in a path (file directory). Generally, packages are used to avoid name conflicts and to write a better supportable code.
Packages are divided into two categories:
- User-defined Packages (create your own packages)
- Built-in Packages (packages from the Java API)
Built-in Packages
The Java API is a library of classes free to use in the Java Development Environment. The library contains components for input, database, programming and so on.
For more details, application programming interface (API) is a list of all classes. These classes are part of the Java development kit (JDK). It includes all Java packages, classes, and interfaces, with their methods, fields, and constructors. These are pre-written classes provide a great amount of functionality to a programmer. A programmer should be aware of these classes and should know how to use them.
Some examples of Java API can be found at Oracle’s website: http://docs.oracle.com/javase/7/docs/api/.
In order to use a class from Java API, one needs to include an import statement at the start of the program.
The API library is divided into packages and classes either import a single or a whole package. When import single package contain class along with its methods and attributes. Also, for a whole package import, contain all the classes that belong to the definite package. To use a class or a package from the library, you need to use the import keyword such as Syntax
import package.name.Class; //for import a single class
import package.name.*; //for import the whole package
Import a Class
When want to use a class, for sample, Scanner class, which is used to get user input, example code:
Example
import java.util.Scanner;
In the example above, java.util is a package, while Scanner is a class of the java.util package. In order to use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation.
What is util?
In Java Package java.util Contains the collections framework, legacy of the collection of various classes, event model, date and time facilities, internationalization, and miscellaneous utility classes, for example, a string tokenizer, a random-number generator, and a bit array.
In our example, we will use the nextLine() method, which is used to read a complete line:
Example
Using the Scanner class to get user input:
import java.util.Scanner;
class Sclass {
public static void main(String[] args) {
Scanner sObj = new Scanner(System.in);
System.out.println("Enter User name");
String userName = sObj.nextLine();
System.out.println("Username is: " + userName);
}
}
OUTPUT
Enter username
hey
Username is: hey
Java Application Programming Interface (API)
For example, in order to use the Scanner class, which allows a program to accept input from the keyboard, one must include the following import statement:
import java.util.Scanner;
The above import statement allows the programmer to use any method listed in the
Scanner class. Another choice for including the import statement is the wildcard option
shown below:
import java.util.*;
This version of the import statement imports all the classes in the API’s java.util package and makes them available to the programmer. If check the API and look at the classes written in the java.util package, you will observe that it includes some of the classes that are used often, such as Arrays, ArrayList, Formatter, Random, and many others. Another Java package that has several commonly used classes is the java.lang package. This package includes classes that are fundamental to the design of Java language. The java.lang package is automatically imported in a Java program and does not need an explicit import statement.
Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes. To import a whole package, end the sentence with an asterisk sign (*). The below example will import all the classes in the java.util package:
Example
import java.util.*;
User-defined Packages
To create own package, Java uses a file system directory to store them. Just like folders on a computer:
Example
└── root
└── mypack
└── MyPackClass.java
To create a package, use the package keyword:
MyPackClass.java
package mypack;
class MyPackClass {
public static void main(String[] args) {
System.out.println("Oh! This is my package !");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Pc Name>javac MyPackClass.java
Then compile the package:
C:\Users\ Pc Name>javac -d. MyPackClass.java
From this path create notepad to practice your java code until expert.
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
UVA Problem 109 ( SCUD Busters) :
This problem is UVA uhunt book chapters (Computational Geometry problem) category problem.
link: http://uva.onlinejudge.org/external/1/109.html
Problem Description:
Find each convex hull for each area then select that convex hull whether a Missile falls inside it.Make aggregation of the area among the selected convex hull.
Problem Solution:
#include <bits/stdc++.h>
#include <math.h>
using namespace std;
int main()
{
double x1,x2,x3,y1,y2,y3,d,e,h,k,r,g,f,c,a;
char selectA,selectB,selectC;
while(scanf(“%lf %lf %lf %lf %lf %lf”,&x1,&y1,&x2,&y2,&x3,&y3)==6)
{
g=((x1*x1+y1*y1-x3*x3-y3*y3)*(y1-y2)-(x1*x1+y1*y1-x2*x2-y2*y2)*(y1-y3))/(2*(x1-x2)*(y1-y3)-2*(x1-x3)*(y1-y2));
f=((x1*x1+y1*y1-x2*x2-y2*y2)*(x1-x3)-(x1*x1+y1*y1-x3*x3-y3*y3)*(x1-x2))/(2*(x1-x2)*(y1-y3)-2*(x1-x3)*(y1-y2));
c=-(x1*x1+y1*y1+2*g*x1+2*f*y1);
h=-g;
k=-f;
r=sqrt(g*g+f*f-c);
if(h<0)
{
selectA=’+’;
h=-h;
}
else
{
selectA=’-‘;
}
if(h==0)
{
selectA=’+’;
h=-h;
}
if(k<0)
{
selectB=’+’;
k=-k;
}
else selectB=’-‘;
if(k==0)
{
selectB=’+’;
k=-k;
}
printf(“(x %c %.3f)^2 + (y %c %.3f)^2 = %.3f^2\n”,selectA,h,selectB,k,r);
if(g<0)
{
selectA=’-‘;
g=-g;
}
else
{
selectA=’+’;
}
if(g==-0)
{
selectA=’+’;
g=-g;
}
if(f<0)
{
selectB=’-‘;
f=-f;
}
else
{
selectB=’+’;
}
if(f==-0){
selectB=’+’;
f=-f;
}
if(c>0) selectC=’+’;
else
{
selectC=’-‘;
c=-c;
}
printf(“x^2 + y^2 %c %.3fx %c% .3fy %c %.3f = 0\n\n”,selectA,2*g,selectB,2*f,selectC,c);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
UVA Problem 476 (Points in Figures Rectangles) :
This problem is UVA uhunt book chapters (Computational Geometry problem) category problem.
Details link: http://uva.onlinejudge.org/external/1/476.html
Problem Description:
This is a straightforward problem.
#include<bits/stdc++.h>
using namespace std;
int main()
{
double x1[10],y1[10],x2[10],y2[10],X,Y;
char ch;
int index=0,p_no=1,fig;
while(1)
{
cin>>ch;
if(ch=='*') break;
cin>>x1[index]>>y1[index]>>x2[index]>>y2[index];
index++;
}
while(scanf("%lf %lf",&X,&Y)==2)
{
if(X==9999.9&&Y==9999.9) break;
bool f=false;
for(fig=0;fig<10;fig++)
{
if(X>x1[fig]&&X<x2[fig]&&Y>y2[fig]&&Y<y1[fig])
{
printf("Point %d is contained in figure %d\n",p_no,fig+1);
f=true;
}
}
if(f==false) printf("Point %d is not contained in any figure\n",p_no);
p_no++;
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
UVA Problem 640 ( Self Numbers): Is a basic UVa problem.
Details link:
Problem Details:
This is a Straightforward problem.
problem solution:
#include <stdio.h>
#include <math.h>
int d[1000010];
int generator(int num)
{
int sum = num;
while(num > 0)
{
sum += num%10;
num /= 10;
}
return sum;
}
int main()
{
int i;
//for(i=1;i<=100;i++)
for(i=1;i<=1000000;i++)
{
if(d[i] == 0)
printf("%d\n",i);
d[generator(i)] = 1;
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
using namespace std;
double arr[1020];
int main()
{
// double P=0,N=0,F,S,T;
int n,i,j;
while(cin>>n && n)
{
double avg=0;
for(i=0;i<n;i++)
{
scanf("%lf",&arr[i]);
avg += arr[i];
}
avg /= n;
double P = 0, N = 0;
for( j = 0; j < n; j++)
{
double res = (long) ((arr[j] - avg) * 100.0) / 100.0;
if(res> 0)
P += res;
else
N += res;
}
N = -N;
if(N>P)
printf("$%.2f\n", N );
else
printf("$%.2f\n",P);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include<bits/stdc++.h>
#define PI acos(-1)
using namespace std;
int main ()
{
double dis_of_sate ,angle,r;
char s[4];
while(cin>>dis_of_sate>>angle>>s) // circle (arc) length s=r*theta. (chord) length = 2*rsin(C/2)
{
if(s[0]=='m') angle/=60;
if(angle>180)
angle=360-angle; // tribhujer kono kon 180 er boro hoy na
r=dis_of_sate + 6440;
angle=PI*angle/180;
printf("%.6lf %.6lf\n",r*angle,2*r*sin(angle/2));
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#include <math.h>
#define pi acos(0.0)
//#define pi 3.1416
int main()
{
double n;
while(scanf("%lf",&n) != EOF )
{
//poligon_inte_angle = (3/5)*180=(108 degree)as figure sides n=5;
//square_inte_angle = (2/4)*90=(63 degree) as figure sides n=4;
printf("%.10lf\n",( n*sin(108*pi/90 ) / sin(63*pi/90) ));//* pi/90
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#include <math.h>
using namespace std;
int main()
{
double a,b,c,ans,s;
while(scanf("%lf %lf %lf",&a,&b,&c) == 3 )
{
s=(a+b+c)/2;
ans=sqrt(s*(s-a)*(s-b)*(s-c));
if(ans>0)
printf("%.3lf\n",ans*(4.0/3.0));
else
{
ans=-1;
printf("%.3lf\n",ans);
}
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#include<iostream>
#include<math.h>
#define pi 2*acos(0.0)
using namespace std;
int main()
{
double n,x,y,a,R,r,A,A1,A2,spect,offic;
int c=0;
while(scanf("%lf %lf",&n,&A)==2 && n>=3)
{
r=sqrt((2*A)/(n*sin(2*pi/n))); //2. Given the radius (circumradius); area(A)=((r^2)nsin(360/n)/2)
R=sqrt( A/(n*tan(pi/n))); //Given the apothem (inradius) area=a^2 ntan(180/n);
//printf("Case %d: %.5lf %.5lf\n",i,R,r);
A1=pi*r*r;
A2=pi*R*R;
spect = A1-A;
offic = A-A2;
printf("Case %d: %.5lf %.5lf\n",c+1,spect,offic);
c++;
}
return 0 ;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#include <cmath>
using namespace std;
int main()
{
int tc;
cin>>tc;
while (tc) {
double ha, hb, hc,a,b,c;//s,s1,a1,b1,c1,a,b,c;
scanf("%lf%lf%lf", &ha, &hb, &hc);
a=1/ha,b=1/hb,c=1/hc;
double s1=(a + b + c);
double a1=(-a + b + c);
double b1=(a - b + c);
double c1=(a + b - c);
double s=s1*a1*b1*c1;
if (s <= 0)
{
printf("These are invalid inputs!\n");
tc--;
}
else if (ha == 0 || hb == 0 || hc == 0)
{
printf("These are invalid inputs!\n");
tc--;
}
else {
printf("%.3f\n", 1 / sqrt(s));
}
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#define PI 2*acos(0)
using namespace std;
int main()
{
int n,tc,i,j;
char t[2];
cin>>tc;
while(tc--)
{
int a,b;
cin>>a;
if(getchar()=='\n')
{
printf("%.4lf\n", (2*a*a*PI)/16);
}
else
{
cin>>b;
printf("%.4lf\n", 2*a*b*PI);
}
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
using namespace std;
int main()
{
int N,a,M,rr;
double x,y;
while(scanf("%d %d",&N,&a)!=EOF && N)
{
bool f = true;
M = 0;
rr = a*a;
for(int i = 0;i<N;++i)
{
scanf("%lf %lf",&x,&y);
f = true;
if((x-0)*(x-0)+(y-0)*(y-0)>rr) //(x1,y1)(x2,y2) bindu gula: (0,0)(0,0)
f = false; //(0,a)(0,a);(a,0)(a,0);(a,a)(a,a)
if((x-0)*(x-0)+(y-a)*(y-a)>rr)
f = false;
if((x-a)*(x-a)+(y-0)*(y-0)>rr)
f = false;
if((x-a)*(x-a)+(y-a)*(y-a)>rr)
f = false;
if(f)
{
M++;
}
}
printf("%.5f\n",(double)M*(a*a)/N);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
#define PI 2*acos(0)
using namespace std;
int main()
{
double d,l,D,L,i,r1,r2;
int tc;
double ans;
cin>>tc;
while(tc--)
{
cin>>D>>L;
d=D/2.0;
l=L/2.0;
r1=sqrt((L/2 * L/2) - (D/2 * D/2));
printf("%.3f\n",PI*r1*l);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include<bits/stdc++.h>
#include <iostream>
#include<math.h>
using namespace std;
int main()
{
double c1,c2,c3,a,b,c,s,ang1,ang2,ang3,arc1,arc2,arc3,area,area1,area2;
int tc;
scanf("%d",&tc);
while(tc--){
scanf("%lf%lf%lf",&c1,&c2,&c3);
a=c1+c2;
b=c2+c3;
c=c1+c3;
s =(a+b+c)/2.0;
area1 = sqrt(s*(s-a)*(s-b)*(s-c));
ang1 = acos((b*b+c*c-a*a)/(2*b*c));
ang2 = acos((c*c+a*a-b*b)/(2*c*a));
ang3 = acos((a*a+b*b-c*c)/(2*a*b));
arc1 =(c3*c3*ang1) ;
arc2 =(c2*c2*ang3);//arc2 =(c3*c3*ang3) ;//arc2 =(c2*c2*ang3) ;
arc3 =(c1*c1*ang2); //arc3 =(c2*c2*ang2);*///arc3 =(c1*c1*ang2);
area2 = (arc1 +arc2 +arc3 )/2;
printf("%.6lf\n",area1-area2);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
using namespace std;
int main()
{
int s,n;
int first_term,last_term;
while (scanf("%d", &s), s != -1)
{
int n_sum =(int)sqrt(2*s);
for (n = n_sum ; n > 0; n--)
{
if ( (2 * s + n - n * n) % (2 * n) == 0 )
{
first_term = (2 * s + n - n * n) / (2 * n);
last_term = first_term + n - 1;
break;
}
}
printf("%d = %d + ... + %d\n", s, first_term, last_term);
// return 0* printf("%d = %d + ... + %d\n", s, a, last_term);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <stdio.h>
long int arr[1000002];
void array ()
{
long int k=0,i;
arr[3]=0;
for( i = 4; i <= 1000000; i++)
{
k = k + ((i-2)/2);
arr[i] = arr[i-1] + k;
}
}
int main()
{
//array();
long int n;
array();
while(scanf("%ld",&n) && n >= 3)
printf("%ld\n",arr[n]);
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <cstdio>
#include <cmath>
using namespace std;
int main()
{
int T,p,q,r,s,t,u;
double lo,hi,mi,f;
while(scanf("%d %d %d %d %d %d",&p,&q,&r,&s,&t,&u)==6)
{
if(p*exp(-1)+q*sin(1)+r*cos(1)+s*tan(1)+t+u>1e-9 || p+r+u<0)
{
printf("No solution\n");
continue;
}
lo=0.0; hi=1.0;
for(int i=0;i<30;i++){
mi=(lo+hi)/2;
f=p*exp(-mi)+q*sin(mi)+r*cos(mi)+s*tan(mi)+t*mi*mi+u;
if(f>0) lo=mi;
else hi=mi;
}
printf("%.4f\n",lo);
}
return 0;
}
by Jesmin Akther | Jan 14, 2019 | Problem Solving, UVa
#include <bits/stdc++.h>
using namespace std;
long double func_tion (long double n)
{
long double i,r = 1;
for ( i = 2; i <= n; i++)
{
r *= i;
}
return r;
}
int main ()
{
long double M, N,r,n,m;
while (cin>>N>>M)
{
if( N==0 and M==0 ) break;
n=(func_tion(N));
m=(func_tion(N-M)*func_tion(M));
r = n/m;
cout << N << " things taken " << M << " at a time is " << setprecision(0) << r << " exactly." << endl;
}
return 0;
}