by Muhammad Harun-Or-Roshid | May 6, 2023 | Laravel
Scoping is one of the superpowers that eloquent grants to developers when querying a model. Scopes allow developers to add constraints
to queries for a given model.
In simple terms laravel scope is just a query, a query
to make the code shorter
and faster
. We can create custom query with relation or anything with scopes.
In any admin project we need to get data using status base, just like active, pending, draft something else. Instead of writing the same query everytime just use scopes and you’re done!
Let’s Start Creating our Scope!
Let’s create a simple with today() scope and it will get only today records.
Step 1: Create Scope in Model
We will add today scope in our post model. So when we query in controller then we will use that scope in laravel model.
app/Post.php
whereDate('created_at', \Carbon\Carbon::today());
}
}
Step 2: Use the scope in your controller
Go to you controller and use the scope by pasting this:
We can also include parameters to make the scope more dynamic!
Step 3: Make the Scope Dynamic by Adding Parameters
Lets go back to the model and add parameters to the scope
app/Post.php
/**
* Scope created awhile ago
*/
public function scopeToday($query)
{
return $query->whereDate('created_at', \Carbon\Carbon::today());
}
/**
* New Dynamic Scope
*/
public function scopeStatus($query, $type)
{
return $query->where('status', $type);
}
Use Both in your controller!
Post::status(1)->today()->get();
Hurray! We have succesfully created our scopes (local scopes) and used it, now use it to your projects and make your code more readable and stable!
I don’t recommend the usage of Global Scopes because it can mess up the readability of queries when you are working with a team, specially if there are new members of the team.
by Muhammad Harun-Or-Roshid | May 10, 2021 | Dart Programming Language
Dart Introduction
Dart is a class-based, garbage-collected, client-optimized, Object-oriented programming (OOP) language for creating fast apps. It can be run on any platform. If you are familiar with an OOP language such as Java you might find many similarities with Dart language.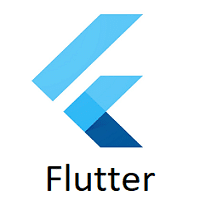
Dart is designed for client development, such as for the web and mobile applications. It is developed by Google and can also be used to build server and desktop applications.
Supported platforms :
Dart language is a very flexible language. It possessed a warm environment in which it can lives. The source code has been written and tested can be deployed in many different ways. For example:
- Stand-alone
- AOT compiled
- Web
- Desktop/Mobile
All the above criteria are discussed in short to make one easy to understand. Firstly, have a look on stand-alone feature what it provides.
Stand-alone: A Java program can’t be run without the Java Virtual Machine (JVM), In the same way as a stand-alone Dart program can’t be executed without the Dart Virtual Machine (DVM). There is a need to download and install the DVM. To execute Dart in a command-line environment DVM is needed. The software development kit (SDK), other than the compiler and the libraries, also offers a series of other development tools, such as:
- the pub package manager.
- dart2js: It is used to compiles Dart code to deployable JavaScript.
- dartdoc: It is the Dart documentation generator.
- dartfmt: This is a code formatter that follows the official style guidelines.
In other words, with the stand-alone way you are creating a Dart program that can only run if the DVM is installed.
AOT compiled:
AOT compilation is very powerful because it natively brings Dart to mobile desktop. The ahead of time compilation is the act of translating a high-level programming language. Like as Dart programing language into native machine code. Basically, starting from the Dart source code you can obtain a single binary file that can execute natively on a certain operating system. With AOT there is NO need to have the DVM installed because at the end you get a single binary file such as an .apk or .aab for Android, an .ipa for iOS, an .exe for Windows. These file can be executed. You’ll end up having a single native binary which doesn’t require a DVM to be installed on the client in order to run the application.
Web:
We blessed having the the dart2js tool, as your Dart project can be “transpiled” into fast. In addition to compact JavaScript code. AngularDart 5 is a performant web app framework used by Google to build some famous websites. The example website are namely “AdSense” and “AdWords”. And it’s powered by Dart!
Desktop/Mobile:
The Just In Time (JIT) technique can be seen as a “real time trans- lation” . The reason for JIT is the compilation happens while the program is executing. It’s a sort of “dynamic compilation” which happens while the program is being used. JIT compilation is combined with the DVM (JIT + VM in the picture). It allows the dispatch of the code dynamically without considering the user’s machine architecture. In this way it is possible to smoothly run and debug the code everywhere. Even though without having to mess up with the underlying architecture.
The draftsbook article with content list are mention here:
- JAVA : Dart is similar feature with JAVA language. Java Classes and objects are the two main aspects of object-oriented programming. The Principles of OOP content are:
-
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
- Data Structure: A data structure is a several way of organizing data in a computer system. In C language different algorithm paradigm with implementation discus in the blog.
- Post Order tree
- Pre Order Tree
- In order Tree
- Binary Search Tree
- Android : Android software development apps can be written using Kotlin, Java, and C++ languages” using the Android software development kit (SDK). This blog contains android feature Navigation drawer implementation and its usage are mention.
- IELTS : IELTS Four module with strategies are written hereby
- Speaking
- listening
- writing
- Reading
- Problem Solving : Problem solution on various online platform tips and solution are given here following platform.
- URI using JAVA languages
- UVA using C, C++ languages
- Timus Using C languages
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (To Carry or not to Carry) 1026:
To Carry or not to Carry is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 15, 2016
* @Time : 4:22:27 PM
*/
public class Uri1026 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
long x, y, answer;
while (sc.hasNext()) {
x = sc.nextLong();
y = sc.nextLong();
answer = x ^ y;
System.out.println(answer);
}
}
}
Please read question carefully.
First of all we have to take two 32 bit decimal numbers as input, and produce an unsigned 32 bit decimal number as the output.
– In above program we take x and y as input and answer as output.
– We have to take x and y value from user and convert them into 32 bit binary.
– And then we have to XOR them and convert to decimal number.
– And after that we put it in answer variable and print it.
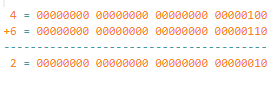
In java programming there is bitwise XOR operator which is ^ . We solved this problem very easily using this bitwise XOR operator.
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Right Area) 1190:
Right Area is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 4:04:11 PM
*/
public class Uri1190 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j > m.length - i - 1 && i < j) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Left Area) 1189:
Left Area is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:57:00 PM
*/
public class Uri1189 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j < m.length - i - 1 && i > j) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Inferior Area) 1188:
Inferior Area is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:45:36 PM
*/
public class Uri1188 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j < i && j > (m.length - i - 1)) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Top Area) 1187:
Top Area is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:32:36 PM
*/
public class Uri1187 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j > i && j < (m.length - i - 1)) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Below the Secundary Diagonal) 1186:
Below the Secundary Diagonal is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:29:44 PM
*/
public class Uri1186 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j > (m.length - i - 1)) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Above the Secundary Diagonal) 1185:
Above the Secundary Diagonal is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:23:06 PM
*/
public class Uri1185 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (j < (m.length - i-1)) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Below the Main Diagonal) 1184:
Below the Main Diagonal is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 3:14:38 PM
*/
public class Uri1184 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (i > j) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Above the Main Diagonal) 1183:
Above the Main Diagonal is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 28, 2016
* @Time : 2:51:24 PM
*/
public class Uri1183 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double m[][] = new double[12][12];
double sum = 0;
int count = 0;
String v = sc.next();
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m.length; j++) {
double x = sc.nextDouble();
m[i][j] = x;
if (i < j) {
sum += x;
count++;
}
}
}
if (v.equals("S")) {
System.out.printf("%.1f\n", sum);
} else {
System.out.printf("%.1f\n", (sum / count));
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Column in Array) 1182:
Column in Array is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 27, 2016
* @Time : 9:20:17 PM
*/
public class Uri1182 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double ars[][] = new double[12][12];
int l = sc.nextInt();
String s = sc.next();
double sum = 0;
if (l >= 0 && l <= 11) {
for (int i = 0; i < ars.length; i++) {
for (int j = 0; j < ars.length; j++) {
ars[i][j] = sc.nextDouble();
if (l == j) {
sum += ars[i][j];
}
}
}
if ("S".equals(s)) {
System.out.printf("%.1f\n", sum);
} else if ("M".equals(s)) {
System.out.printf("%.1f\n", (sum / 12));
}
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Line in Array) 1181:
Line in Array is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @E-mail : [email protected]
* @Date : Oct 27, 2016
* @Time : 8:57:36 PM
*/
public class Uri1181 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double ars[][] = new double[12][12];
int l = sc.nextInt();
String s = sc.next();
double sum = 0;
if (l >= 0 && l <= 11) {
for (int i = 0; i < ars.length; i++) {
for (int j = 0; j < ars.length; j++) {
ars[i][j] = sc.nextDouble();
if (l == i) {
sum += ars[i][j];
}
}
}
if("S".equals(s)){
System.out.printf("%.1f\n",sum);
}else if("M".equals(s)){
System.out.printf("%.1f\n",(sum/12));
}
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Lowest Number and Position) 1180:
Lowest Number and Position is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 27, 2016
* @Time : 8:30:34 PM
*/
public class Uri1180 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int value = 0, position = 0;
int n = sc.nextInt();
if (1 < n && n < 1000) {
int ars[] = new int[n];
for (int i = 0; i < ars.length; i++) {
ars[i] = sc.nextInt();
if(value>ars[i]){
value=ars[i];
position= i;
}
}
System.out.println("Menor valor: "+value+"\n"+"Posicao: "+position);
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Array Fill IV) 1179:
Sum of Consecutive Even Numbers is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 27, 2016
* @Time : 10:58:46 PM
*/
public class Uri1179 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int par[] = new int[5];
int impar[] = new int[5];
int p = 0;
int im = 0;
for (int i = 0; i < 15; i++) {
int x = sc.nextInt();
if (x % 2 == 0) {
par[p] = x;
p++;
} else {
impar[im] = x;
im++;
}
if (p == 5) {
int c = 0;
while (c < p) {
System.out.println("par[" + c + "] = " + par[c]);
c++;
}
p = 0;
}
if (im == 5) {
int d = 0;
while (d < im) {
System.out.println("impar[" + d + "] = " + impar[d]);
d++;
}
im = 0;
}
if (i == 14) {
int d = 0;
while (d < im) {
System.out.println("impar[" + d + "] = " + impar[d]);
d++;
}
int c = 0;
while (c < p) {
System.out.println("par[" + c + "] = " + par[c]);
c++;
}
}
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Sum of Consecutive Even Numbers) 1159:
Sum of Consecutive Even Numbers is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 22, 2016
* @Time : 11:34:44 AM
*/
public class Uri1159 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while (true) {
int x = sc.nextInt();
int count = 0, total = 0;
if (x == 0) {
break;
} else {
while (count < 5) {
if (x % 2 == 0) {
total += x;
count++;
}
x++;
}
System.out.println(total);
}
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Sum of Consecutive Odd Numbers III) 1158:
Sum of Consecutive Odd Numbers III is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 21, 2016
* @Time : 11:12:29 PM
*/
public class Uri1158 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for (int i = 0; i < n; i++) {
int x = sc.nextInt();
int y = sc.nextInt();
int count = 0,total = 0;
while(y>count){
if(x%2!=0){
total+=x;
count++;
}
x++;
}
System.out.println(total);
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (Divisors I) 1157:
Divisors I is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
import java.util.Scanner;
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 21, 2016
* @Time : 11:06:28 PM
*/
public class Uri1157 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for (int i = 1; i <= n; i++) {
if(n%i==0){
System.out.println(i);
}
}
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (S Sequence II) 1156:
S Sequence II is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 21, 2016
* @Time : 10:53:21 PM
*/
public class Uri1156 {
public static void main(String[] args) {
double total = 0;
int count = 1;
for (int i = 1; i <= 39; i+=2) {
double s = (double)i/(double)(count);
total+=s;
count*=2;
}
System.out.printf("%.2f\n",total);
}
}
by Muhammad Harun-Or-Roshid | Feb 7, 2019 | Problem Solving, URI
URI Problem (S Sequence) 1155:
S Sequence is a basic problem on URI online judge for novice problem solver.
You can find details on this Link.
/**
* @Author : Muhammad Harun-Or-Roshid
* @Date : Oct 21, 2016
* @Time : 10:27:56 PM
*/
public class Uri1155 {
public static void main(String[] args) {
double total = 0;
for (int i = 1; i <= 100; i++) {
double p = 1 / (double) i;
total += p;
}
System.out.printf("%.2f\n", total);
}
}