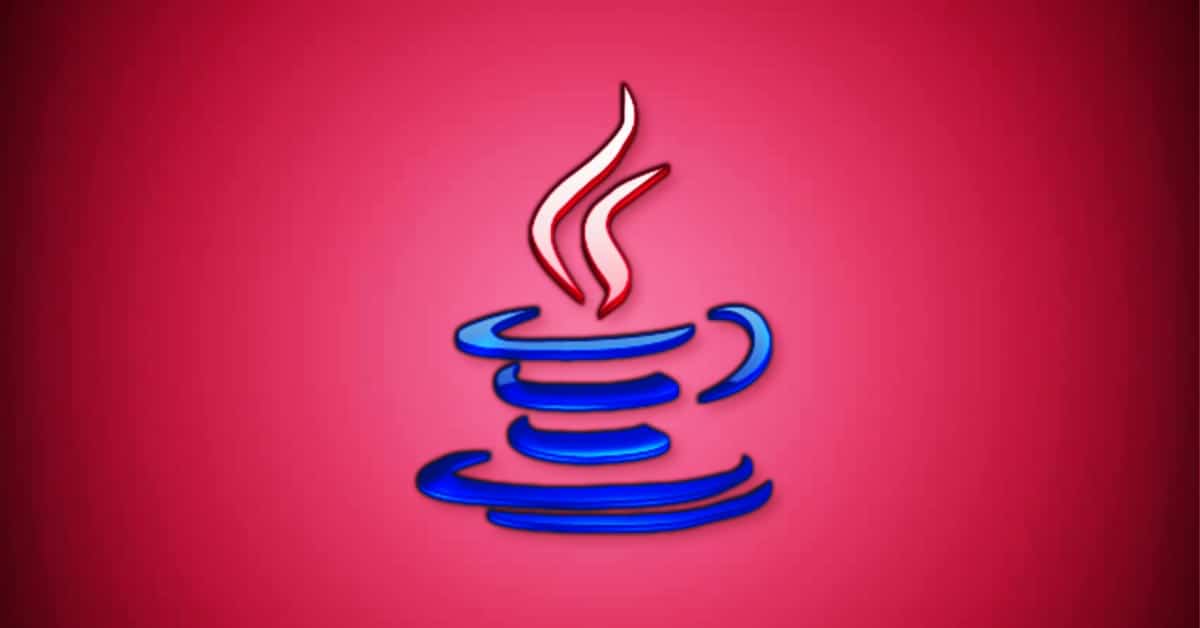
Part 22: Java Lambda Expressions with real time Example
Java Lambda Expressions
Lambda Expressions in java were added in Java 8. When you implemented an anonymous simple class, such as an interface which contains only one method, then the syntax of anonymous classes may seem vogue and unclear. In these scenario, trying to pass an argument to another method, In this time when someone clicks a button Lambda expressions enable to do the action, to act as functionality as method argument, or code as data.
In short saying, A lambda expression is a short block of code which takes in parameters and returns a value. This expressions are alike to methods, though they do not need a name and they can be implemented right in the body of a method.
Syntax
The simplest lambda expression contains a single parameter and an expression:
parameter -> expression
To use more than one parameter, wrap them in parentheses:
(parameter1, parameter2) -> expression
Expressions are limited to use. They have to immediately return a value, and they cannot contain variables, assignments or statements for example if or for. To do more complex operations, a code block can be used with curly braces. If the lambda expression needs to return a value, then the code block should have a return statement.
(parameter1, parameter2) -> { code block }
Using Lambda Expressions
Lambda expressions are usually passed as parameters to a function:
Example
Use a lamba expression in the ArrayList’s forEach() method to print every item in the list:
import java.util.ArrayList; public class Example{ public static void main(String[] args) { ArrayList<Integer> numbers = new ArrayList<Integer>(); numbers.add(5); numbers.add(9); numbers.add(8); numbers.add(1); numbers.forEach( (n) -> { System.out.println(n); } ); } }
OUTPUT:
9
8
1
Lambda expressions can be stored in variables(if variable type is an interface) which has only one method. The lambda expression should have the similar number of parameters and the same return type as that method. Java has many of these kinds of interfaces built in, such as the Consumer interface (found in the java.util package) used by lists.
Example
Use Java’s Consumer interface to store a lambda expression in a variable:
import java.util.ArrayList; import java.util.function.Consumer; public class Main { public static void main(String[] args) { ArrayList<Integer> numbers = new ArrayList<Integer>(); numbers.add(5); numbers.add(9); numbers.add(8); numbers.add(1); Consumer<Integer> method = (n) -> { System.out.println(n); }; numbers.forEach( method ); } }
OUTPUT:
9
8
1
To use a lambda expression in a method, the method should have a parameter with a single-method interface as its type. Calling the interface’s method will run the lambda expression:
Example
Create a method which takes a lambda expression as a parameter:
interface StringFunction { String run(String str); } public class Example{ public static void main(String[] args) { StringFunction exclaim = (s) -> s + "!"; StringFunction ask = (s) -> s + "?"; printFormatted("Hello", exclaim); printFormatted("Hello", ask); } public static void printFormatted(String str, StringFunction format) { String result = format.run(str); System.out.println(result); } }
Lambdas expressions and the Single Method Interface
As Functional programming is frequently used to implement event listeners. Event listeners in Java are often defined as Java interfaces with a single method. Here is a single method interface example:
public interface StateChangeListener { public void onStateChange(State oldState, State newState); }
This Java interface defines a single method which is called when the state changes or whatever is being observed.
In Java 7 you would have to implement this interface due to listen for state changes. Suppose, a class called StateOwner which can register state event listeners. Here is an example:
public class StateOwner { public void addStateListener(StateChangeListener listener) { ... } }
In Java 7 could add an event listener using an anonymous interface implementation, like this:
StateOwner stateOwner = new StateOwner(); stateOwner.addStateListener(new StateChangeListener() { public void onStateChange(State oldState, State newState) { // do something with old and new state. } });
First a StateOwner instance is created. Next an anonymous implementation of the StateChangeListener interface is added as listener on the StateOwner instance.
In Java 8, can add an event listener using a Java lambda expression, like this:
StateOwner stateOwner = new StateOwner(); stateOwner.addStateListener( (oldState, newState) -> System.out.println("State changed") );
Here the lambda expressions is this part:(oldState, newState) -> System.out.println(“State changed”)
The lambda expression is matched against the parameter type of the addStateListener() method parameter. If the matches the parameter type (in this case the StateChangeListener interface) , then the lambda expression is turned into a function that implements the same interface as that parameter.