by Jesmin Akther | Dec 27, 2018 | JAVA
Key Point on Overriding vs Overloading:
Overriding is a run-time concept while overloading is a compile-time concept. In any object-oriented programming language(OOP), The ability of a subclass to override a method. This process allows a class to inherit from a super class whose behavior is frequently similar in nature and then to modify behavior as needed. Use the “@Override” annotation to instructs the compiler that intend to override a method in the superclass. If the compiler detects that the method does not exist in one of the super classes, then it will generate an error.
Besides in Java OOP, two or more methods may have the same name if they differ in parameters such as different number of parameters, different types of parameters, or both. In These methods are called overloaded methods and this feature is called method overloading. These Overloading methods have the same name but accept different arguments. Method Overloading in Java with examples briefly discuss in below. Method Overloading feature allows a class to have more than one method having the same name.
Step by step overriding vs. overload in JAVA features with real example is given below.
Overriding Features:
- Same method name.
- Same parameter list.
Overriding allows a child class to provide a specific implementation of a method that is already provided its parent class.
In below example Learn is parent class and Teach is child class and share() method is specific implementation of method between these class.
class Learn{
public void share(){
System.out.println("class learn method share");
}
}
class Teach extends Learn{
public void care(){
System.out.println("class Teach method care");
}
public void share(){
System.out.println("class Teach method share");
}
}
Here Learn and Teach both class uses same method same name,same perameter share().
Overloading Features:
- Same method name.
- Different parameter list.
class Learn{
public void share(int n){
System.out.println("class learn method share");
}
public void share(int n1,int n2){
System.out.println("class Teach method share");
}
}
During compilation time Compiler know they are different because they have different method signature.Two method signature are like:
share(int)
share(int,int)
if both method have
- same method name.
- same number of arguments.
- and same type of arguments.
like below then compiler show compile error.
share(int n)
share(int n)
Draftsbook is a learning base blog. The blog share Article series based on Subject. For example:
- JAVA : JAVA is a object-oriented programming(OOP) language. This language classes and objects are the two main aspects of OOP. The blog content discuss below principles of OOP:
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
- Data Structure: Data structure refers a several way of organizing data in a computer system.
- Android : Android software development apps can be written using Kotlin, Java, and C++ languages” using the Android software development kit (SDK). This blog contains android feature Navigation drawer implementation and its usage are mention.
- IELTS : IELTS Four module with strategies are written hereby
5.Problem Solving : Problem solution on various online platform tips and solution are given here following platform.
by Jesmin Akther | Dec 27, 2018 | JAVA
Method Signature in Java:
Firstly need to know what is java method? why to use in java?
Java method refers, a method which is a block of code which only runs when it is called. Here data can pass as parameters into a method. Methods are used to perform certain actions, and they are also known as functions.
Use:
This is mainly use to frequent use a same function by call that is reuse a function.
To create a method must be declared within a class. It is followed by parentheses () with the name of the method. Java provides some pre-defined methods, such as System.out.println(), though can also create own user define methods to perform secific actions:
Example
Create a method inside Tree:
public class Tree{
static void treeMethod() {
// code to be executed
}
}
Example Explained
- treeMethod() is the name of the method.
- static means that the method belongs to the Main class and not an object of the Tree class.
- void means that this method does not have a return value.
Now with another example brief it shortly:
Next need to know what is method signature and it’s declaration. It is refers to a method-name to parameter-list before body part of a java class.
An example of method declaration:
public double showValue(int types,double area)
{
//to do
}
So in method declaration uses components are:
modifiers: It controls the access level,
Modifier |
Description |
public |
The public use in code which mean it is accessible for all classes. |
private |
This Modifier refers the code is only accessible within the declared class |
default |
The code is only accessible in the same package. This is used when don’t want to specify a modifier. |
protected |
The code is accessible in the same package and subclasses. |
return type: A return type may be a primitive type. For instance int, float, double, a reference type or void type(returns nothing),
method name: A method name should follow the camel case. First letter of the first word should be small and the first letters of the remaining words should be capital,
parameter list in parenthesis: Parameters act as variables inside the method.
public class Main
{
static void mMethod(String first_name)
{
System.out.println(first_name );
}
public static void main(String[] args)
{
mMethod("Harun");
mMethod("Roshid");
}
}
Output:
Harun
Roshid
exception list: Java Built-in exceptions are suitable to explain certain error situations. It also supports users to define their own exceptions.,
- ArithmeticException
This exception is thrown in code when an exceptional condition has occurred in an arithmetic operation.
- ArrayIndexOutOfBoundsException
It is thrown to indicate that an array has been accessed either negative or greater than or equal to the size of the array.
the method body: The method body is where all of the action of a method takes place,
enclosed between braces: Start to end body of a Java class .
Among these components(six total) only two comprises the method signature.
- a method name,
- parameter list in parenthesis
Following the example the method signature :
showValue( int types, double area)
That means, method signature is part of the method declaration , combination of the method name and the parameter list. Overloading method signature get emphasis to its ability to write methods that have the same name but accept different parameters.
Through the method signatures , Java compiler can discern the difference between the methods at compilation time.
Method Signature Examples:
public double showValue(int types,double area,float result) {
//to do
}
The method signature in the above example is showValue (int, double, float)
public double showValue(String name) {
//to do
}
The Java compiler will let us add another method like the above example because its method signature is different showValue(String) in this case.
To Call a Method :
we discuss before in Java, write the method’s name followed by two parentheses () and a semicolon;
In the following example, treeMethod() is used to print a text (the action), when it is called:
Example
Inside main, call the treeMethod() method:
public class Tree {
static void treeMethod() {
System.out.println("hello executed treeMethod!");
}
public static void main(String[] args) {
treeMethod();
}
}
// Outputs “hello executed treeMethod!”
The method can be called multiple time. Above code main method rewrite for this code:
public static void main(String[] args) {
treeMethod();
treeMethod();
treeMethod();
}
}
// Here treeMethod() call three times and everytime shows same output
Java Method Parameters and Arguments:
Value can be passed to methods as parameter. Parameters act as variables inside the method.
Parameters are specified after the method name, inside the parentheses. You can add as many parameters as you want, just separate them with a comma.
public class Tree {
static void myMethod(String languageName) {
System.out.println(languageName+ " language");
}
public static void main(String[] args) {
myMethod("Python");
myMethod("C");
myMethod("C++");
}
}
// Python language
// C language
// C++ language
Java can support Multiple Parameters. You can have as many parameters as you like:
Example
public class Tree{
static void myMethod(String languageName, (String version) {
System.out.println(languageName + " is a" + age);
}
public static void main(String[] args) {
myMethod("Python", "language");
myMethod("C", "language");
myMethod("C++", "language");
}
}
// Python is a language
// C is a language
// C++ is a language
Draftsbook is a learning base blog. The blog share Article series based on Subject. For example:
-
- JAVA : JAVA is a object-oriented programming(OOP) language. This language classes and objects are the two main aspects of OOP. The blog content discuss below principles of OOP:
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
- Data Structure: Data structure refers a several way of organizing data in a computer system.
- Android : Android software development apps can be written using Kotlin, Java, and C++ languages” using the Android software development kit (SDK). This blog contains android feature Navigation drawer implementation and its usage are mention.
- IELTS : IELTS Four module with strategies are written hereby
5.Problem Solving : Problem solution on various online platform tips and solution are given here following platform.
by Jesmin Akther | Dec 27, 2018 | Interview Programming
C:
Reverse a given string without using build in function
#include <stdio.h>
#include<string.h>
int main()
{
char string1[100],string2[100];
char s[]="Learn and share blog";
int i,j,k,count;
for(count = 0; s[count] != '\0'; count++);
for(j=count-1,i=0 ;j>=0; j--)
{
string1[i]=s[j];
i++;
}
string1[i]='\0';
printf("reverse string is : %s",string1);
return 0;
}
output:
reverse string is : golb erahs dna nraeL
by Jesmin Akther | Dec 27, 2018 | Interview Programming
Length of string without build in function.
#include <stdio.h>
#include<string.h>
int main()
{
char s[]="Learn and share blog";
int count;
for(count = 0; s[count] != '\0'; count++);
printf("String Length : %d\n", count);
return 0;
}
output:
String Length : 20
by Jesmin Akther | Dec 27, 2018 | Interview Programming
Count length of string using recursion(without build in function).
#include<stdio.h>
#include <string.h>
int length_count(char *line)
{
static int count = 0;
if(*line != NULL)
{
count++;
length_count(++line);
}
return count;
}
int main()
{
char str[] ="Learn and share blog";
int count=0;
printf("The string length is :\t");
count=length_count(str);
printf("%d",count);
return 0;
}
Output:
The string length is : 20
by Jesmin Akther | Dec 27, 2018 | Interview Programming
Reverse a string using recursion.
Procedure:
#include<stdio.h>
#include <string.h>
void reverse(char *line)
{
if(*line)
reverse(line+1);
printf("%c",*line);
}
int main()
{
char str[] ="Learn and share blog";
printf("The reverse string is :\t");
reverse(str);
return 0;
}
Output:
The reverse string is : golb erahs dna nraeL
by Jesmin Akther | Dec 24, 2018 | JAVA
Interface in Java
Interface is a similar kind of java class. It can be called as collection of abstract methods or another way of achieving abstract method as it contains empty bodies. It can declare as: interface <any-name>{}.
Example
/* interface */
interface Box
{
/*interface method does not have a body */
public void boxHieght();
/*interface method does not have a body */
public void run();
}
Interface Feature:
- Class can contains variable, method etc. Similarly for interface.
- Like class interface have method, variable but these method does not contain any body part. This is main difference between interface and class.
- When we use any class, this can extends only one class. But a class can use multiple interface and all the interface method will be used as override. These override method can’t be contained any ambiguous problem.
Class which are used interface need to use implements such as
Class Example implements Shadow {} package interfaccepackage;
public interface Shadow{
/*implicitly public abstract method*/
void manshadow();
/*implicitly public static final*/
int x = 100;
}
1. Here we show that the method is contain void but implicitly here the method actually used as public abstract if we do not write down in manshadow() method.
2. Variable x is not only int but also public static final either we write it or not.
When we declare any interface and any class implements this interface then compiler show that the interface has implementations and method has override. Below we use a class named as Example, where we implements Shadow interface.
When any class implements any interface must be override all the interface methods in the class otherwise will compilation error.
package interfaccepackage;
public Class Example implements Shadow {
int y;
public int noOflegs();
return 10;
}
public void printmn(){
System.out.println(“Class method println statements : “);
}
public static void main(String args[]){
Example ex = new Example ();
System.out.println(ex.y);
System.out.println(x);
System.out.println(ex.noOflegs());
ex.manshadow();
ex.printmn();
}
@Override
public void manshadow(){
System.out.println(“Interface method manshadow statements prints: “);
}
}
noOflegs(), printmn() are Example class method. Manshadow () method is override and this is a public method due to interface this method is default access method. When we use method of interface we need to override the method less restricted in the class. In Example class public is less restricted than default so we override manshadow () method as public.
Like Example class more class can be implements Shadow() interface and can override all the methods as user requirements
Output of the program
run:
0
100
10
Interface method manshadow statements prints:
Class method println statements :
0 is for the glabal variable y’s default initialization value.
100 interface variable x’s value
10 is noOflegs() method of Example class value.
First println is Example class
Second println is Override method
Interface is mainly used for polymorphism. Multiple classes can implements multiple interface. Besides multiple interface can extends multiple interfaces. But a java class cannot extends multiple interface because java support single inheritance.
These interface feature are explain with example below:
Interface TextA:
public interface TextA{
public abstract void print();
public static final int var=0;
}
Interface TextB:
public interface TextB{
void print();
}
There is two interface TextA and TextB both contains same method but there will be no ambiguity problem. But if we use it in C++ then there will show ambiguity problem. Now we check ambiguity when we implements these interfaces in a class named Neon {}
public Class Noon implements TextA, TextB{
void method(){
System.out.println(“Outside method in java : “);
}
public static void main(String args[]){
Neon n = new Neon();
n.print();
n.method();
}
@Override
public void print(){
System.out.println(“Outside method in java: “);
}
}
Here is the override method print(). If we click print() method ‘I’ key this will show implements from both interface TextA and TextB and work properly what we use in this method. That means interface has no interface ambiguity problem. Another uses of interface is class Neon can be abstract so print() method need not mandatory override. But if we wish we can use override. There is a problem of using abstract class because In abstract class no object can be created. Similarly
- Interface no object can be created.
- An interface can extends another interface
public interface TextA extends TextB
{
public abstract void bprint();
public static final int var=0;
}
That is an interface can used another interface using extends.
Another feature of interface is marker interface. In this interface no method or variable included for example Cloneable(). This interface send special information to JVM. Again @Override in one kind of interface.
Cloneable() interface
package java.lang;
public interface Cloneable{
}
Cloneable() is default interface. We can implement user defined empty interface too.
package java.lang;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(value = (ElementType.METHOD))
@Retention(value =RetentionPolicy.SOURCE )
public @interface Override{
}
More other tutorials on JAVA in Draftsbook Article series on :
- JAVA : Dart is similar feature with JAVA language. Java Classes and objects are the two main aspects of object-oriented programming. The Principles of OOP are:
-
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
by Jesmin Akther | Dec 24, 2018 | JAVA
Nested class in JAVA with EXAMPLES
Nested class if we declare one or more classes in a class then these classes are known as nested class.
Java Allows two category of nested class
- Static nested class
- Non Static nested class or inner class.
i) Member inner class: When declare any class inside a class but outside a method
ii) Anonymous inner class: this class uses for interface and abstract
iii) Local inner class: this class is more restricted class than others. This class used inside method.
Why use nested class? When we declare a private variable in a class others class can’t use this variable. So the purpose of nested class is hiding or encapsulate.
Inner class or non-static class can use outer class variable as global variable.
Member inner class example:
-
public class Outerclass {
private int data=30;
class Inner{
void printline(){
System.out.println("inner class Printline");
System.out.println("Outer class data variable value: "+data);
}
}
void display(){
Outerclass.Inner inner = new Outerclass.Inner();
inner.printline();
}
public static void main(String args[]){
Outerclass outer = new Outerclass();
outer.display();
}
}
Following the program Inner class use as member inner class. To display any line in the Inner class and to print outer class variable, here need outer class object in main method.
Line 10 can be rewritten as Inner inner = new Inner(). Keep in mind we can’t create Inner class object in main method because main method is a static method. In outer class create display method where need to create inner class object to print inner class method.
When outer.display() called then inner class method printline() is run.
Output:
run:
inner class Printline
30
BUILD SUCCESSFUL (total time: 0 seconds)
Outer class variable value run as globally, In case of Inner class variable or method need to create inner class object that already used in the example.
Anonymous inner class: This type of inner class most important. This inner class used in interface and abstract. As interface and abstract can’t create any object so inner class is the best use for interface and inner class.
Interface consist of ‘able’ ,e.g default interface Cloneable()
Now example for anonymous inner class
-
interface Enable{
void screen();
}
public class TestClass{
public static void main(String args[]){
Enable e = new Enable() {
@Override
public void screen() {
System.out.println("Nice and Simple");
}
};
e.screen();
}
}
Following the example Enable is an interface there contains a method void screen(). To implement the method there needs to be @Override following
-
interface Enable{
void screen();
}
public class TestClass implements Enable{
public static void main(String args[]){
}
@Override
public void screen() {
System.out.println("Using implements Enable interface");
}
}
We can use Enable interface by using implements. When implements Enable interface this TestClass show message for Override method of interface.
So anonymous inner class can be used as inner class or by implements interface.
As this class known as anonymous we can rewrite the inner class like
-
new Enable() {
@Override
public void screen() {
System.out.println("Using implements Enable interface");
}
}.screen();
Here this inner class means obj.method() where object is anonymous. This anonymous inner class can be used for interface similarly for the abstract class. We know interface is implicitly public abstract where no method has any body part. For the abstract class user need to declare class and method as abstract. Example anonymous inner class for abstract class:
-
abstract interface newinterface
{
abstract void eat();
}
public class NewClass {
public static void main(String args[])
{
new newinterface(){
@Override
public void eat()
{
System.out.println("Sweet food");
}
}.eat();
}
}
Here this inner class means obj.method() where object is anonymous and the class is abstract.
Local inner class:
A class that is created inside a method is known as local inner class. If you want to invoke the methods of local inner class, you must instantiate this class inside the method.
1) Local inner class cannot be invoked from outside the method.
2) Local inner class cannot access non-final variable.
Example of local inner class:
public class LocalInner {
private int data = 30;
void localmethod()
{
class Inner
{
void message()
{
System.out.println("Local variable value: "+data);
}
}
Inner inner = new Inner();
inner.message();
}
public static void main(String args[])
{
LocalInner obj = new LocalInner();
obj.localmethod();
}
}
In main method when obj.localmethod(); is called local inner method called to run the inner class method that is inner.message(); is called.
Another example
public class LocalInner {
private int data = 30;
void localmethod() {
int x=2;
class Inner {
void message() {
System.out.println("Local variable value: "+data +" " +x);
} }
Inner inner = new Inner();
inner.message(); }
public static void main(String args[])
{
LocalInner obj = new LocalInner();
obj.localmethod();
} }
Here we declare a local variable x (line 5) in the inner class and want to invoke message() method then need to declare x as final. Example
void localmethod() {
final int x=2; class Inner{
void message() {
System.out.println("Local variable value: "+data+" " +x);
} }
Above all if local variable does not final,local variable can’t invoke the local inner class.
Static Nested Class;
Example:
public class NewClass1 {
static int a =1;
static class Inner{
void message()
{
System.out.println("Simple statements " +a);
} static void display()
{ System.out.println("Display statements");
} }
public static void main(String args[]) {
Inner n = new Inner();
n.message(); // as message() is non-static so need to create object to call the method.
Inner.display(); //display() method is static so can be called by class ,no need create object here. } }
Nested Interface: Interfaces can be nested, by default nested Interfaces are public and static (implicitely) Nested Interface program: public interface Displable { void msg(); interface Eatable{ void eat(); } }
When we want to use eat() method in class the program would be:
class Exinterface implements Displable.Eatable{
@Override
public void eat() {
System.out.print(" Mango ");
}
}
public class NestedInterface{
public static void main(String args[]){
Exinterface ex = new Exinterface();
ex.eat();
}
}
In this program we implements outer interface class with inner interface to use eat() method.
When we want to use msg() method in class the program would be:
class Exinterface implements Displable{
@Override
public void msg() {
System.out.print(" This is a Nested Interface example ");
}
}
public class NestedInterface{
public static void main(String args[]){
Exinterface ex = new Exinterface();
ex.msg();
}
}
by Jesmin Akther | Dec 24, 2018 | JAVA
Java abstract class with Example
When we declare any class with abstract then this class known as abstract class. Abstract class can method can be used as abstract and non-abstract. In Interface all methods implicitly public abstract and we know abstract methods can’t be any body part.
Example:
abstract class First
{
abstract void printA();
void printAB()
{
System.out.println("This is non-abstract method of abstract class First");
}
}
Here printA() is an abstract method where printAB() is a non-abstract method. So, abstract class provide both abstract and non-abstract method.
Now, another class (e.g. class Second) wants to use this abstract class then that class need to extends the First class. The class Second can’t extends more than one class because the class maintain single inheritance. After extends class “First” need to override method printA() because this is an abstract method. printAB() method can be or can’t be override because this is optional and this method is not abstract. For the interface class, when another class need to use interface class then all the method interface mandatorily need to override.
Example:
class Second extends First
{
@Override void printA()
{
System.out.println("This is override method abstract class First");
}
}
In this part, printA() method is override as default same as class “First” printA() method. If class “First” is an interface then printA() method mendatorilly override as public.
Keep in mind abstract class has not any object references directly. If we want to create any abstract class object then we need to create inner class with override method of abstract class.
public class AbstractDemo {
public static void main(String args[]){
Second second = new Second();
second.printA();
second.printAB();
}
}
Here create object of second class to call the methods. As class”First” is abstract so we did not create any object of this class.
So abstract class main advantages are need not to mandatorily override all the methods in abstract class. Only which class are need in frequently we declare these methods as abstract latterly we use these methods as override and change methods body part as required.
Extra features of abstract class are given below using an program:
abstract class Initfirst
{
int dim1,dim2;
Initfirst(int d1, int d2)
{
this.dim1 = d1;
this.dim2 =d2;
}
abstract int sum();
abstract int mul();
}
class Initsecond extends Initfirst
{
public Initsecond(int d1, int d2)
{
super(d1, d2);
}
@Override int sum()
{
return (dim1 + dim2);
}
@Override int mul()
{
return (dim1 * dim2);
}
}
class Inittheird extends Initfirst
{
public Inittheird(int d1, int d2)
{
super(d1, d2);
}
@Override int mul()
{
return (dim1 * dim2);
}
@Override int sum()
{
return (dim1 + dim2);
}
}
public class ClassDemo
{
public static void main(String args[])
{
Initsecond initsecond = new Initsecond(2,1);
Inittheird inittheird = new Inittheird(4,5);
Initfirst initfirst; initfirst = initsecond;
System.out.println("Sum is : "+initfirst.sum() + " " +"Mul is : "+initfirst.mul());
initfirst = inittheird;
System.out.println("Sum is : "+initfirst.sum() + " " +"Mul is : "+initfirst.mul());
}
}
Following the program abstract class Initfirst contructor can not called directly like
Initfirst initfirst = new Initfirst(1,2); But Initfirst class subclass can be called directly by creating object.
Initsecond and Inittheird both extends Initfirst so Initfirst construct called by super() which is used both subclasses in Initsecond and Inittheird.
In main method both subclasses object is created. As abstract class Initfirst can not be called directly so we can call it initialize as “ Initfirst “any_name”; Follwing program Initfirst initfirst;
So we can make call by writing Initfirst
As, Initfirst = initsecond;
Initfirst = inittheird;
by Jesmin Akther | Dec 22, 2018 | Interview Programming
Prime Number:
A positive number whose divisors only 1 and the number itself (among all the positive numbers) are known as prime number.
Example: 1,2,3,5,7,11.
Among positive even number only 2 is the prime number rest of all the prime numbers are odd(positive) number.
#include<stdio.h>
int main()
{
int number,i,j,primeNumber,low=1,high=100;
printf(" Prime Numbers are: \n");
for(j=low;j<=high;j++){
primeNumber=0;
for(i=2;i<=j/2;i++){
if(j%i==0){
primeNumber++;
break;
}
}
if(primeNumber==0 && j !=1)
printf("%d\t ",j);
}
return 0;
}
output:
Prime Numbers are:
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
53 59 61 67 71 73 79 83 89 97
by Jesmin Akther | Dec 22, 2018 | Interview Programming
Prime Number:
A positive number whose divisors only 1 and the number itself (among all the positive numbers) are known as prime number.
Example: 1,2,3,5,7,11.
Among positive even number only 2 is the prime number rest of all the prime numbers are odd(positive) number.
#include<stdio.h>
int main()
{
int number,i,j,primeNumber=0;
printf("please Enter a Number:\n");
scanf("%d",&number);
for(i=2;i<=number/2;i++){
if(number%i==0)
primeNumber++;
break;
}
if(primeNumber==0 && number!=1)
printf("%d is a Prime Number\n",number);
else
printf("%d is not a Prime Number\n",number);
return 0;
}
output:
please Enter a Number:
5
5 is a Prime Number.
by Jesmin Akther | Dec 21, 2018 | Interview Programming
#include <stdio.h>
int main()
{
while(1)
printf("Hello World\n");
return 0;
}
Here condition 1 means during condition checking time while loop return condition is always true.
Output:
Hello World
Hello World
Hello World
Hello World
.
.
.
.
.
by Jesmin Akther | Dec 14, 2018 | Interview Programming
Armstrong Number:
A number is refer as Armstrong number if the numbers each digits cubic sum is equivalent to that number.
Example: 153 = (1)^3 + (5)^3 + (3)^3.
#include <stdio.h>
int main()
{
int number,i,j,sum,reminder,tempo,min=1,max=250;
//printf("Enter a number\n");
//scanf("%d",&number);
for(number=min;number<=max;number++)
{
tempo =number;
sum=0;
while(tempo != 0)
{
reminder = tempo%10;
sum=sum+(reminder*reminder*reminder);
tempo=tempo/10;
}
if(sum==number)
{
printf("%d is a armstrong number\n",number);
}
}
return 0;
}
Output:
1 is a armstrong number.
153 is a armstrong number.
by Jesmin Akther | Dec 14, 2018 | Interview Programming
C program to check a number is Armstrong number or not.
Armstrong Number:
A number is refer as Armstrong number if the numbers each digits cubic sum is equivalent to that number.
Example: 153 = (1)^3 + (5)^3 + (3)^3.
C program:
#include <stdio.h>
int main()
{
int number,i,j,sum,reminder,tempo;
printf("Enter a number\n");
scanf("%d",&number);
tempo =number;
sum=0;
while(number != 0)
{
reminder = number%10;
sum=sum+(reminder*reminder*reminder);
number=number/10;
}
if(sum==tempo)
{
printf("%d is a armstrong number\n",tempo);
}
else
{
printf("%d is not a armstrong number\n",tempo);
}
return 0;
}
OUTPUT:
Enter a number:
153
153 is a armstrong number.
by Jesmin Akther | Dec 13, 2018 | Interview Programming
Check wheather a number is Perfect number or not.
Perfect number are summation of divisors of a number exclude the number ownself.
Eample 6,28.
Lets check 28. From 1 to 27 we need to check which number can divide 28.
then sum of all those number whose are divisors of 28.
28 = 1,2,7,14(divisors).
Now make sum of all divisors
1+2+7+14 = 28
so 28 is perfect number.
Again 6 divisors are 1,2,3
so, 1+2+3 = 6 which is also perfect number.
C program:
#include <stdio.h>
int main()
{
int number,perfect_number,start=1,sum=0;
printf("Input Any Number: \n");
scanf("%d",&number);
while(start<number)
{
if(number%start == 0)
sum= sum+start;
start ++;
}
if(sum ==number)
{
printf("%d is perfect number\n",number);
}
else
{
printf("%d is not perfect number\n",number);
}
return 0;
}
by Jesmin Akther | Sep 17, 2018 | Data Structure
Post-order:
Post-order traverse need to traverse left, then right then root. Array, Linked List, Queues, Stacks, etc. are linear traversal where have only one logical way to traverse them. Though trees can be traversed in different ways. That Traversals:
- In-order (Left, Root, Right)
- Preorder (Root, Left, Right)
- Postorder (Left, Right, Root)
For Post-order traverse need to traverse left, then right then root.
Following figure Post -Order traverse 2, 7, 9.

tree
Algorithm for Post-order traverse.
Input tree, root.
Step 1: Traverse left sub tree like call Pre-order (tree, root. left).
Step 2: Traverse right sub tree like call Pre-order (tree, root. right).
Step 3: visit root node.
Now, Traverse the following tree by following program.
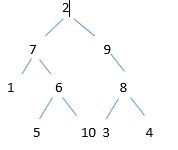
tree
Traverse : 1, 5, 10, 6, 7, 3, 4, 8, 9, 2.
Recent Articles on Binary Tree in the blog Topic :
- Introduction Of Binary Tree
- Traversals such as
Implementation Process for Post-order traverse:
- 1st need to create node struct where data, *left , *right declared;
- 2nd need to create new node function which take an item and return new node, where firstly need i) memory allocation same as datatype size. If failed to memory allocation show error or assign data into new node,if left or right child no value then put them as NULL.
- 3rd to add child left and right need two function both take two input one for node pointer another for left or right child assigned.
- 4th create create_tree() function by create_node ,add_left_child() and right_child() function. This function return root.
- 5th Now use post_order() recursion function, if left child not null call post_order() recursively for left node,Again if right child not null call post_order()recursively for right node, root print.
- 6th main() function receive root data or value will print.
Implementation Using C :
A
#include <stdio.h>
typedef struct node Node;
struct node
{
int data;
Node *left;
Node *right;
};
Node *create_node (int item)
{
Node *new_node = (Node *) malloc(sizeof(Node));
if(new_node == NULL)
{
printf(“Error ! Could not create an Error\n”);
exit(1);
}
new_node -> data = item;
new_node -> left= NULL;
new_node ->right =NULL;
return (new_node);
}
void add_left_child( Node *node, Node *child )
{
node->left =child;
}
void add_right_child( Node *nodee, Node *child )
{
nodee->right =child;
}
Node *create_tree()
{
Node *two = create_node(2);
Node *seven=create_node(7);
Node *nine=create_node(9);
add_left_child(two,seven);
add_right_child(two,nine);
Node *one = create_node(1);
Node *six = create_node(6);
add_left_child(seven,one);
add_right_child(seven,six);
Node *five=create_node(5) ;
Node *ten = create_node(10);
add_left_child(six,five);
add_right_child(six,ten);
Node *eight = create_node(8);
add_right_child(nine,eight);
Node *three=create_node(3);
Node *four=create_node(4);
add_left_child(eight,three);
add_right_child(eight,four);
return two;
}
void post_order(Node *node)
{
if(node->left != NULL)
{
post_order(node->left);
}
if(node->right != NULL)
{
post_order(node->right);
}
printf(“%d “,node->data);
}
struct Node node;
int main()
{
Node *root =create_tree();
post_order(root);
printf(” “);
return 0;
}
OUTPUT: 1 5 10 6 7 3 4 8 9 2
Data Structure more articles
by Jesmin Akther | Sep 17, 2018 | Data Structure
In-order Traversal of a Tree.
In-order traversal is very commonly used on binary search trees(BST).Tt returns values from the underlying set in order, according to the comparator set up of the binary search tree. Array, Linked List, Queues, Stacks, etc. are linear traversal where have only one logical way to traverse them. Though trees can be traversed in different ways. That Traversals:
- In-order (Left, Root, Right)
- Preorder (Root, Left, Right)
- Postorder (Left, Right, Root)
In-order:
For In-order traverse need to traverse left, then root, then right.

tree
Following figure In-order traverse 7,2,9.
Algorithm for In-order traverse.
Input tree, root.
Step 1: Traverse left sub tree like call In-order (tree, root. left).
Step 2: visit root node.
Step 3: Traverse right sub tree like call In-order(tree, root. right).
Now, Traverse the following tree by following program.
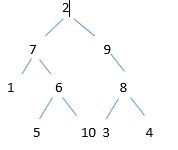
tree
Traverse : 1,7,5,6,10,2,9,3,8,4.
Recent Articles on Binary Tree in the blog Topic :
- Introduction Of Binary Tree
- Traversals such as
Implementation Process:
1st need to create node struct where data, *left , *right declared;
2nd need to create new node function which take an item and return new node, where firstly need i) memory allocation same as datatype size. If failed to memory allocation show error or assign data into new node, if left or right child no value then put them as NULL.
3rd to add child left and right need two function both take two input one for node pointer another for left or right child assigned.
4th create create_tree() function by create_node ,add_left_child() and right_child() function. This function return root.
5th Now use in_order() recursion function, if left child not null call in_order() recursively for left node. root print , then Again if right child not null call in_order()recursively for right node.
6th main() function receive root data or value will print.
Program:
#include <stdio.h>
typedef struct node Node;
/* A binary tree node has data, pointer to left child and a pointer to right child */
struct node
{
int data;
Node *left;
Node *right;
};
Node *create_node (int item)
{
Node *new_node = (Node *) malloc(sizeof(Node)); /* create storage*/
if(new_node == NULL) { printf(“Error ! Could not create an Error\n”);
exit(1);
}
new_node -> data = item;
new_node -> left= NULL;
new_node ->right =NULL;
return (new_node);
}
void add_left_child( Node *node, Node *child )
{
node->left =child;
}
void add_right_child( Node *nodee, Node *child )
{
nodee->right =child;
}
Node *create_tree()
{
Node *two = create_node(2);
Node *seven=create_node(7);
Node *nine=create_node(9);
add_left_child(two,seven);
add_right_child(two,nine);
Node *one = create_node(1);
Node *six = create_node(6);
add_left_child(seven,one);
add_right_child(seven,six);
Node *five=create_node(5) ;
Node *ten = create_node(10);
add_left_child(six,five);
add_right_child(six,ten);
Node *eight = create_node(8);
add_right_child(nine,eight);
Node *three=create_node(3);
Node *four=create_node(4);
add_left_child(eight,three);
add_right_child(eight,four);
return two;
}
/* print tree nodes according to the in order traversal. */
void in_order(Node *node)
{
if(node->left != NULL)
{
in_order(node->left);
}
printf(“%d\n”,node->data);
if(node->right != NULL)
{
in_order(node->right);
}
}
//struct Node node; int main()
{
/* Given a binary tree, to print its nodes according to the in order traversal. */
Node *root =create_tree();
in_order(root);
printf(“\n”);
return 0;
}
Data Structure more articles
- PostOrder traverse C Program For Pre_Order traversal in a Tree.
- In-order traverse C program In_order Traversal of a Tree.
- Post order traversal
Post-order traverse of a Tree with C program.
by Jesmin Akther | Sep 17, 2018 | Data Structure
Pre-order traversal:
Pre-order traverse need to traverse root, then left, then right. Array, Linked List, Queues, Stacks, etc. are linear traversal where have only one logical way to traverse them. Though trees can be traversed in different ways. That Traversals:
- In-order (Left, Root, Right)
- Preorder (Root, Left, Right)
- Postorder (Left, Right, Root)
For Pre-order traverse need to traverse root, then left, then right.

tree
Following figure Pre–order traverse 2, 7, 9.
- Input tree, root.
- Step 1: visit root node.
- Step 2: Traverse left sub tree like call Pre-order (tree, root. left).
- Step 3: Traverse right sub tree like call Pre-order (tree, root. right).
Now, Traverse the following tree by following program.
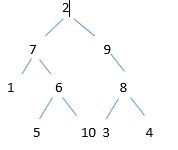
tree
Traverse : 2,7,1,6,5,10,9,8,3,4.
Recent Articles on Binary Tree in the blog Topic :
- Introduction Of Binary Tree
- Traversals such as
C Implemetation Process:
- 1st need to create node struct where data, *left , *right declared;
- 2nd need to create new node function which take an item and return new node, where firstly need i) memory allocation same as datatype size.
If failed to memory allocation show error or assign data into new node,if left or right child no value then put them as NULL.
- 3rd to add child left and right need two function both take two input one for node pointer another for left or right child assigned.
- 4th create create_tree() function by create_node ,add_left_child() and right_child() function. This function return root.
- 5th Now use pre_order() recursion function, root print first, then if left child not null call pre_order() recursively for left node. Again if right child not null call pre_order()recursively for right node.
- 6th main() function receive root data or value will print.
C Program For Pre-order traversal in a Tree.
#include <stdio.h>
/*create a data type node */
typedef struct node Node;
struct node{
int data;
Node *left;
Node *right;
};
/*Create each node and corresponding child.Allocate memory for new node if new node empty. show error either keep item data variable,initialize left and right child into NULL then return new */
Node *create_node (int item) {
Node *new_node = (Node *) malloc(sizeof(Node));
if(new_node == NULL)
{
printf(“Error ! Could not create an tree\n”);
exit(1);
}
new_node -> data = item;
new_node -> left= NULL;
new_node ->right =NULL;
return (new_node);
}
/*add left node corresponding parent node */
void add_left_child( Node *node, Node *child ) {
node->left =child;
}
/*add right node corresponding parent node */
void add_right_child( Node *node, Node *child ) {
node->right =child;
}
/*create tree for node (2,7),(2,9),(7,1),(7,6),(6,5),(6,10),(9,8),(8,3),(8,4)*/
Node *create_tree() {
Node *two = create_node(2);/*create root node: 2 */
Node *seven=create_node(7);/*create root node: 7 */
Node *nine=create_node(9);/*create root node: 9 */
add_left_child(two,seven);/*create root node: 7 */
add_right_child(two,nine);/*create root node: 9 */
Node *one = create_node(1); /*create parent node 1 */
Node *six = create_node(6);/*create parent node:6 */
add_left_child(seven,one);/*add parent node seven left:1*/
add_right_child(seven,six);/*add parent seven node right:6*/
Node *five=create_node(5) ;/* similar process rest of all*/
Node *ten = create_node(10); add_left_child(six,five);
add_right_child(six,ten);
Node *eight = create_node(8); add_right_child(nine,eight);
Node *three=create_node(3);
Node *four=create_node(4);
add_left_child(eight,three); add_right_child(eight,four);
return two;/* return root node*/
} /*print root node 2; */
void pre_order(Node *node){
printf(“%d\n”,node->data);
if(node->left != NULL){
pre-order(node->left);}
else if(node->right != NULL){
pre-order(node->right);}}
int main() {
Node *root =create_tree();
pre_order(root);
/*printf(“%d”,root->data);*/
printf(” “);
return 0;
}
Data Structure more articles
- PostOrder traverse C Program For Pre_Order traversal in a Tree.
- In order traverse C program In_order Traversal of a Tree.
- Post order traversal Post-order traverse of a Tree with C program.
by Jesmin Akther | Jun 1, 2018 | Data Structure
Binary Tree:
A binary tree each node has at most two children generally referred as left child and right child from root node.
Components of Each node:
- Pointer to left subtree
- Pointer to right subtree
- Data element
The topmost node in the tree is called the root. An empty tree is represented by NULL pointer.
A tree which has two nodes corresponding their parent node is known as binary tree.
Binary tree term as two types.
1) Full Binary tree.
A tree which has two nodes corresponding their parent node is known as full binary tree.
2) Complete Binary tree.
3) A tree which has left nodes corresponding their parent node is known as complete binary tree.
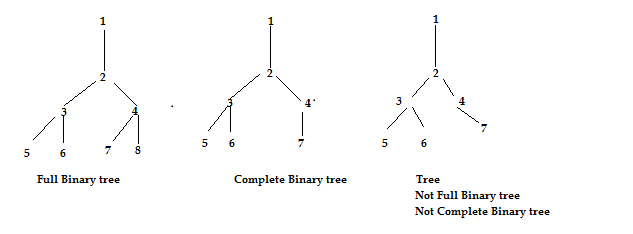
Common Terminologies of Binary Tree:
- Root: Topmost node in a tree.
- Parent: Every node (excluding a root) in a tree is connected by a directed edge from exactly one other node. This node is called a parent.
- Child: A node directly connected to another node when moving away from the root.
- Leaf/External node: Node with no children.
- Internal node: Node with atleast one children.
- Depth of a node: Number of edges from root to the node.
- Height of a node: Number of edges from the node to the deepest leaf. Height of the tree is the height of the root.
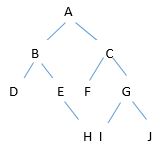
Binary Tree
In the binary tree root node is A. The tree has 10 nodes with 5 internal nodes A,B,C,E,G and 5 external nodes D,F,H,I,J. The height of the tree is 3. B is the parent of D and E while D and E are children of B.
C implementation Process:
1st need to create node struct where data, *left , *right declared;
2nd need to create new node function which take an item and return new node, where firstly need i) memory allocation same as datatype size.
If failed to memory allocation show error or assign data into new node,if left or right child no value then put them as NULL.
3rd to add child left and right need two function both take two input one for node pointer another for left or right child assigned.
4th create create_tree() function by create_node ,add_left_child() and right_child() function. This function return root.
5th main() function receive root data or value will print.
C Program For binary tree.
#include <stdio.h>
/*create a data type node */
typedef struct node Node;
struct node{
int data;
Node *left;
Node *right;
};
/*Create each node and corresponding child.Allocate memory for new node if new node empty. show error either keep item data variable,initialize left and right child into NULL then return new */
Node *create_node (int item)
{
Node *new_node = (Node *) malloc(sizeof(Node));
if(new_node == NULL)
{
printf(“Error ! Could not create an tree\n”);
exit(1);
}
new_node -> data = item;
new_node -> left= NULL;
new_node ->right =NULL;
return (new_node);
}
/*add left node corresponding parent node */
void add_left_child( Node *node, Node *child )
{
node->left =child;
}
/*add right node corresponding parent node */
void add_right_child( Node *node, Node *child )
{
node->right =child;
}Node *seven=create_node(7);/*create root node: 7 */
Node *nine=create_node(9);/*create root node: 9 */
add_left_child(two,seven);//*create root node: 7 */
add_right_child(two,nine);/*create root node: 9 */
Node *one = create_node(1); /*create parent node 1 */
Node *six = create_node(6);/*create parent node:6 */
add_left_child(seven,one);/*add parent node seven left:1*/
add_right_child(seven,six);/*add parent seven node right:6*/
Node *five=create_node(5) ;/* similar process rest of all*/
Node *ten = create_node(10); add_left_child(six,five);
add_right_child(six,ten);
Node *eight = create_node(8); add_right_child(nine,eight);
Node *three=create_node(3);
Node *four=create_node(4);
add_left_child(eight,three); add_right_child(eight,four);
return two;/* return root node*/
} /*print root node 2; */
int main()
{
Node *root =create_tree();
printf(“%d”,root->data);
return 0;
}
Recent Articles on Binary Tree in the blog Topic :
- Introduction Of Binary Tree
- Traversals such as